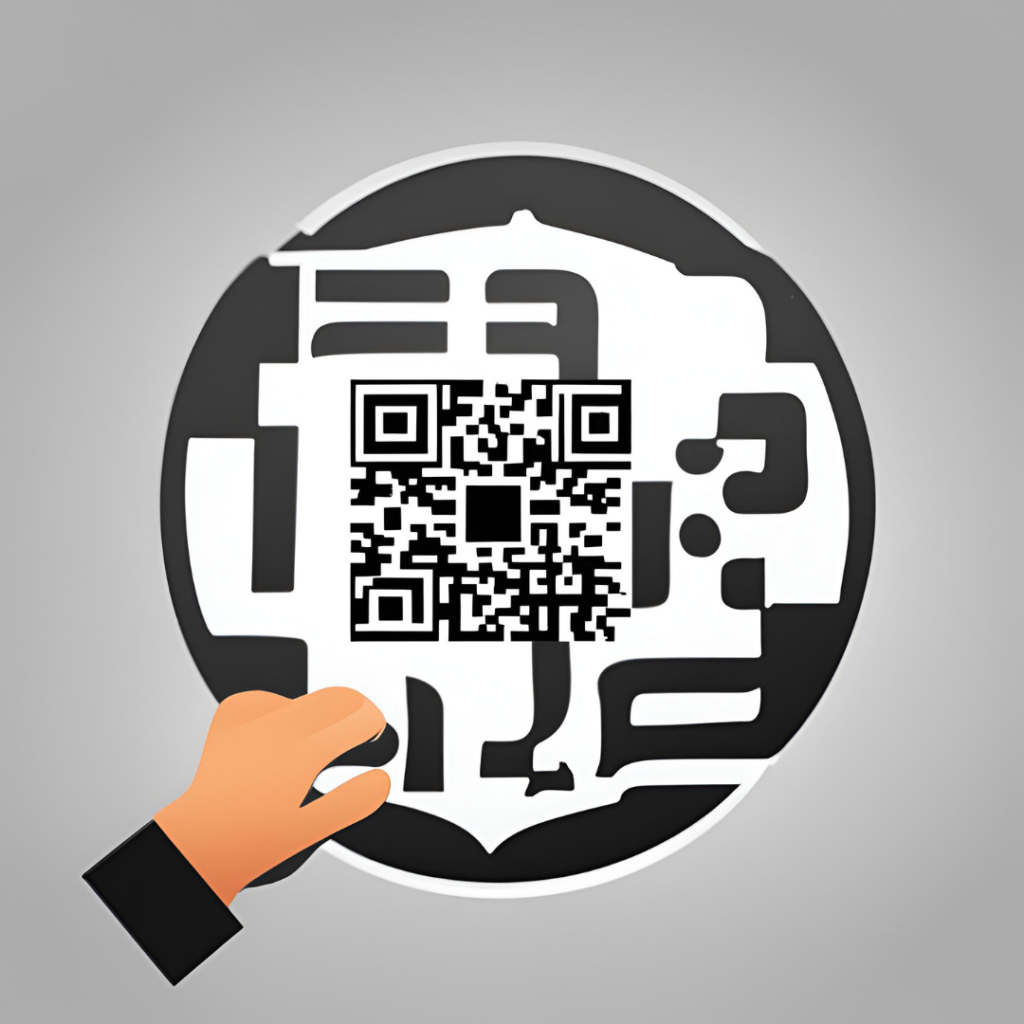
Creating PDFs dynamically in web applications is a common requirement. One popular library for generating PDFs in the .NET environment is iTextSharp. This powerful tool allows for extensive PDF manipulations, including the addition of QR codes. However, developers might encounter issues when working with QR codes, especially regarding format recognition. In this blog post, we'll discuss how to generate PDFs with QR codes using iTextSharp in ASP.NET and address a specific issue we recently encountered and resolved.
The Problem
Recently, while working on a project that required embedding QR codes in PDFs, we ran into an issue where the QR code format was unrecognized. This issue disrupted our workflow as the QR code, essential for our application, couldn't be read or processed correctly.
The Solution
After some investigation and troubleshooting, we identified that the problem stemmed from the way the QR code was being handled in the PDF. The solution was to convert the QR code into an image format before embedding it into the PDF. This approach ensured that the QR code retained its integrity and could be recognized correctly.
Here’s a step-by-step guide on how we implemented this solution using iTextSharp's BarcodeQRCode class.
Step-by-Step Guide
- Install iTextSharp
First, ensure that you have the iTextSharp library installed in your ASP.NET project. You can install it via NuGet Package Manager with the following command:
Install-Package itextsharp
- Generate the QR Code
Use the BarcodeQRCode class to generate the QR code. Convert this QR code into an image format to avoid recognition issues.
using iTextSharp.text;
using iTextSharp.text.pdf;
// URL or text to be encoded in the QR code
string caseQRUrl = “https://example.com/your-case-url”;
// Create the QR code
BarcodeQRCode qrcode = new BarcodeQRCode(caseQRUrl, 1, 1, null);
// Convert the QR code to an image
Image img = qrcode.GetImage();
- Create the PDF and Embed the QR Code
With the QR code image ready, you can now create a PDF document and embed the QR code into it.
// Create a Document object
Document document = new Document();
// Set the file path where the PDF will be saved
string pdfPath = Server.MapPath(“~/GeneratedPDFs/QRCodePDF.pdf”);
// Create a PdfWriter instance
PdfWriter.GetInstance(document, new FileStream(pdfPath, FileMode.Create));
// Open the document for writing
document.Open();
// Add the QR code image to the document
document.Add(img);
// Close the document
document.Close();
- Handle the Response
If you need to send the PDF as a response to the client's browser, you can modify the code to stream the PDF directly.
Response.ContentType = “application/pdf”;
Response.AddHeader(“Content-Disposition”, “attachment; filename=QRCodePDF.pdf”);
Response.OutputStream.Write(File.ReadAllBytes(pdfPath), 0, File.ReadAllBytes(pdfPath).Length);
Response.Flush();
Response.End();
Conclusion
Generating PDFs with QR codes in ASP.NET using iTextSharp can be straightforward. However, you might encounter issues with QR code recognition if not handled correctly. By converting the QR code to an image format using the BarcodeQRCode class, you can ensure that the QR code is embedded correctly and remains readable.
This approach resolved our issue and can help you avoid similar problems in your projects. Happy coding!